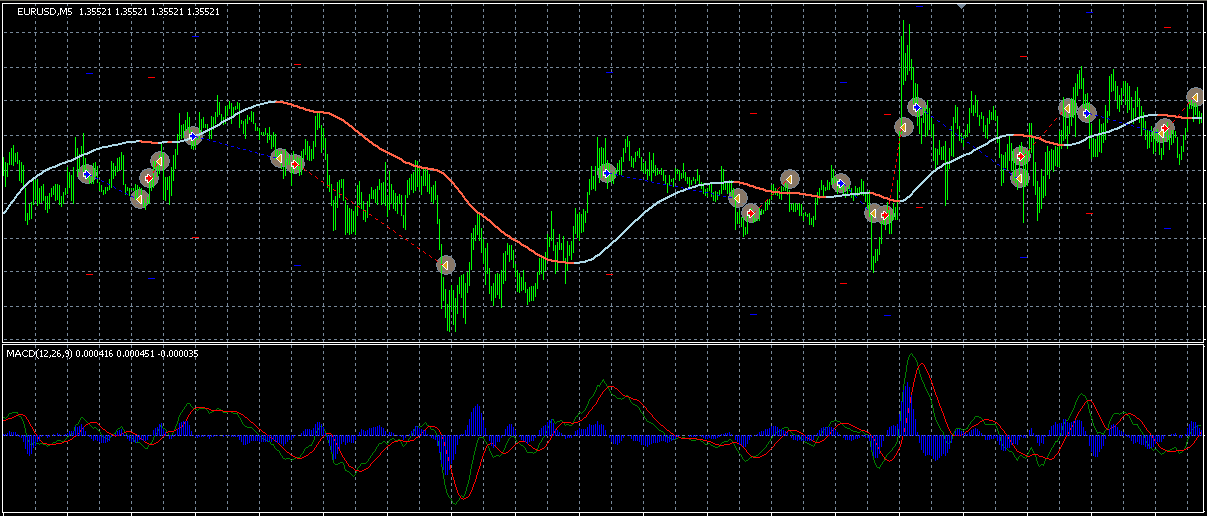
//+------------------------------------------------------------------+
//| Renko.mq4 |
//| Copyright © 2014, AM2 |
//| http://www.forexsystems.biz |
//+------------------------------------------------------------------+
#property copyright "Copyright © 2011, AM2"
#property link "http://www.forexsystems.biz"
#property description "Slope Directional Line expert advisor"
#define MAGICMA 20141111
//--- Inputs
extern double StopLoss = 500; // стоп лосс ордера
extern double TakeProfit = 500; // тейк профит ордера
extern double Lots = 0.1; // объем позиции
//----
extern int period = 90; // период средней Slope Directional Line
extern int method = 2; // метод средней(SMA=0,EMA=1,SMMA=2,LWMA=3)
extern int price = 0; // цена по которой строится средняя
//----
extern int FastPeriod = 12; // период быстрого макди
extern int SlowPeriod = 26; // период медленного макди
extern int SignalPeriod = 9; // период сигнального макди
//----
extern int MACDAC = 1; // включение макди(1-включен,0-отключен)
bool buy=true,sell=true;
//+------------------------------------------------------------------+
//| Check for open order conditions |
//+------------------------------------------------------------------+
void CheckForOpen()
{
int res;
//--- go trading only for first tiks of new bar
if(Volume[0]>1) return;
//--- get Moving Average
double SlopeBlue=iCustom(Symbol(),0,"Slope",period,method,price,0,0);
double SlopeRed=iCustom(Symbol(),0,"Slope",period,method,price,1,0);
double MACDGreen=iCustom(Symbol(),0,"MACDTraditional",FastPeriod,SlowPeriod,SignalPeriod,1,0);
double MACDRed=iCustom(Symbol(),0,"MACDTraditional",FastPeriod,SlowPeriod,SignalPeriod,0,0);
//--- sell conditions
if(MACDAC==1)
{
if(SlopeBlue>SlopeRed && MACDGreen<MACDRed && sell==true)
{
res=OrderSend(Symbol(),OP_SELL,Lots,Bid,3,Bid+StopLoss*Point,Bid-TakeProfit*Point,"",MAGICMA,0,Red);
sell=false;
buy=true;
return;
}
//--- buy conditions
if(SlopeRed>SlopeBlue && MACDGreen>MACDRed && buy==true)
{
res=OrderSend(Symbol(),OP_BUY,Lots,Ask,3,Ask-StopLoss*Point,Ask+TakeProfit*Point,"",MAGICMA,0,Blue);
buy=false;
sell=true;
return;
}
}
else
{
if(SlopeBlue>SlopeRed && sell==true)
{
res=OrderSend(Symbol(),OP_SELL,Lots,Bid,3,Bid+StopLoss*Point,Bid-TakeProfit*Point,"",MAGICMA,0,Red);
sell=false;
buy=true;
return;
}
//--- buy conditions
if(SlopeRed>SlopeBlue && buy==true)
{
res=OrderSend(Symbol(),OP_BUY,Lots,Ask,3,Ask-StopLoss*Point,Ask+TakeProfit*Point,"",MAGICMA,0,Blue);
buy=false;
sell=true;
return;
}
}
//---
}
//+------------------------------------------------------------------+
//| Check for close order conditions |
//+------------------------------------------------------------------+
void CheckForClose()
{
//--- go trading only for first tiks of new bar
if(Volume[0]>1) return;
//--- get Moving Average
double SlopeBlue=iCustom(Symbol(),0,"Slope",period,method,price,0,0);
double SlopeRed=iCustom(Symbol(),0,"Slope",period,method,price,1,0);
//---
for(int i=0;i<OrdersTotal();i++)
{
if(OrderSelect(i,SELECT_BY_POS,MODE_TRADES)==false) break;
if(OrderMagicNumber()!=MAGICMA || OrderSymbol()!=Symbol()) continue;
//--- check order type
if(OrderType()==OP_BUY)
{
if(SlopeBlue>SlopeRed)
{
if(!OrderClose(OrderTicket(),OrderLots(),Bid,3,White))
Print("OrderClose error ",GetLastError());
}
break;
}
if(OrderType()==OP_SELL)
{
if(SlopeRed>SlopeBlue)
{
if(!OrderClose(OrderTicket(),OrderLots(),Ask,3,White))
Print("OrderClose error ",GetLastError());
}
break;
}
}
//---
}
//+------------------------------------------------------------------+
//| OnTick function |
//+------------------------------------------------------------------+
void OnTick()
{
//--- check for history and trading
if(Bars<100 || IsTradeAllowed()==false)
return;
//--- calculate open orders by current symbol
if(OrdersTotal()<1) CheckForOpen();
else CheckForClose();
//---
}
//+------------------------------------------------------------------+
AM2