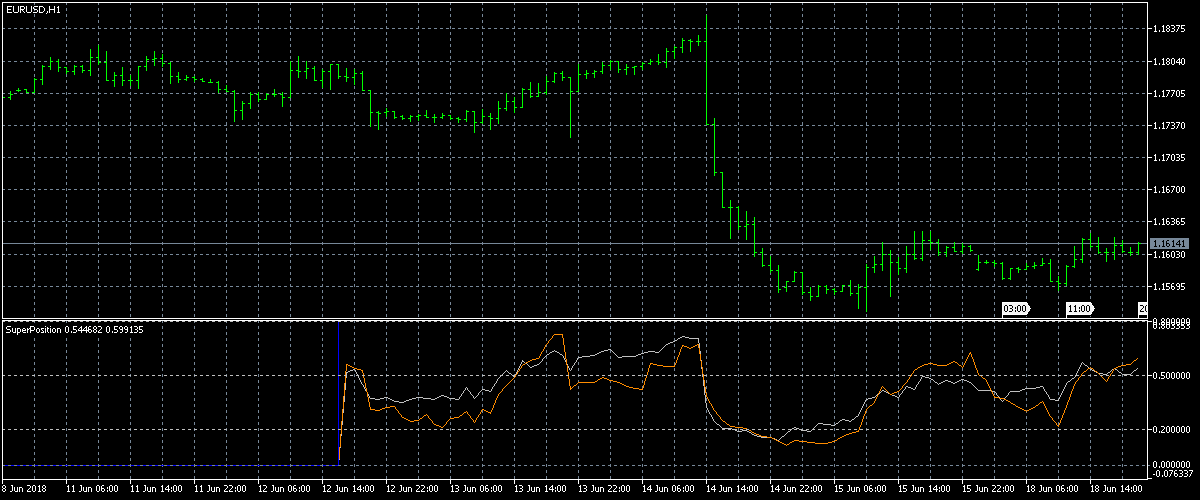
сейчас загвоздка в том что не знаю как пользоваться функциями библиотеки: <MovingAverages.mqh>
ExponentialMAOnBuffer(rates_total,prev_calculated,0,FastMA,SPBuffer,FastMABuffer);
ExponentialMAOnBuffer(rates_total,prev_calculated,0,SlowMA,SPBuffer,SlowMABuffer);
нужно переписать этот код:
for(i=0; i<limit; i++)
{
FastMABuffer[i]=iMAOnArray(SPBuffer,Bars,FastMA,0,MODE_LWMA,i);
SlowMABuffer[i]=iMAOnArray(SPBuffer,Bars,SlowMA,0,MODE_LWMA,i);
DiverBuffer[i]=iMAOnArray(TmpDiverBuff,Bars,FastMA,0,MODE_LWMA,i)*2;
}
пока так:
//+------------------------------------------------------------------+
//| SuperPosition.mq5 |
//| Copyright 2018, AM2 |
//| http://www.forexsystems.biz |
//+------------------------------------------------------------------+
#property copyright "Copyright 2018, AM2"
#property link "http://www.forexsystems.biz"
#property version "1.00"
#property indicator_separate_window
#property indicator_buffers 15
#property indicator_plots 5
//---- plot RSI
#property indicator_label1 "RSI" // Название линии, отображаемое во всплывающей подсказе при наведении на линии указателя мыши.
#property indicator_type1 DRAW_LINE // Тип буфера
#property indicator_color1 Silver // Цвет линии
#property indicator_style1 STYLE_SOLID // Стиль
#property indicator_width1 1 // Толщина линии
//---- plot DeMarker
#property indicator_label2 "DeMarker" // Название линии, отображаемое во всплывающей подсказе при наведении на линии указателя мыши.
#property indicator_type2 DRAW_LINE // Тип буфера
#property indicator_color2 DarkOrange // Цвет линии
#property indicator_style2 STYLE_SOLID // Стиль
#property indicator_width2 1 // Толщина линии
//---- plot SPB
#property indicator_label3 "SPB" // Название линии, отображаемое во всплывающей подсказе при наведении на линии указателя мыши.
#property indicator_type3 DRAW_LINE // Тип буфера
#property indicator_color3 Black // Цвет линии
#property indicator_style3 STYLE_SOLID // Стиль
#property indicator_width3 1 // Толщина линии
//---- plot FastMA
#property indicator_label4 "FastMA" // Название линии, отображаемое во всплывающей подсказе при наведении на линии указателя мыши.
#property indicator_type4 DRAW_LINE // Тип буфера
#property indicator_color4 Red // Цвет линии
#property indicator_style4 STYLE_SOLID // Стиль
#property indicator_width4 1 // Толщина линии
//---- plot SlowMA
#property indicator_label5 "SlowMA" // Название линии, отображаемое во всплывающей подсказе при наведении на линии указателя мыши.
#property indicator_type5 DRAW_LINE // Тип буфера
#property indicator_color5 Blue // Цвет линии
#property indicator_style5 STYLE_SOLID // Стиль
#property indicator_width5 1 // Толщина линии
//-----Level lines
#property indicator_level1 -0.20
#property indicator_level2 0.20
#property indicator_level3 0.50
#property indicator_level4 0.80
#include <MovingAverages.mqh>
//---- indicator parameters
input int RSI=10;
input int DeMarker=10;
input int SPStep=4;
input int FastMA=10;
input int SlowMA=18;
double RSIBuffer[];
double DeMarkerBuffer[];
double SPBuffer[];
double FastMABuffer[];
double SlowMABuffer[];
double TmpDiverBuff[];
double DiverBuffer[];
double RSI0[];
double RSI1[];
double RSI2[];
double RSI3[];
double DM0[];
double DM1[];
double DM2[];
double DM3[];
//+------------------------------------------------------------------+
//| Custom indicator initialization function |
//+------------------------------------------------------------------+
int OnInit()
{
//--- indicator buffers mapping
SetIndexBuffer(0,RSIBuffer,INDICATOR_DATA);
SetIndexBuffer(1,DeMarkerBuffer,INDICATOR_DATA);
SetIndexBuffer(2,RSI0,INDICATOR_CALCULATIONS);
SetIndexBuffer(3,RSI1,INDICATOR_CALCULATIONS);
SetIndexBuffer(4,RSI2,INDICATOR_CALCULATIONS);
SetIndexBuffer(5,RSI3,INDICATOR_CALCULATIONS);
SetIndexBuffer(6,DM0,INDICATOR_CALCULATIONS);
SetIndexBuffer(7,DM1,INDICATOR_CALCULATIONS);
SetIndexBuffer(8,DM2,INDICATOR_CALCULATIONS);
SetIndexBuffer(9,DM3,INDICATOR_CALCULATIONS);
SetIndexBuffer(10,SPBuffer,INDICATOR_DATA);
SetIndexBuffer(11,TmpDiverBuff,INDICATOR_CALCULATIONS);
SetIndexBuffer(12,FastMABuffer,INDICATOR_DATA);
SetIndexBuffer(13,SlowMABuffer,INDICATOR_DATA);
//---
return(INIT_SUCCEEDED);
}
//+------------------------------------------------------------------+
//| Custom indicator iteration function |
//+------------------------------------------------------------------+
int OnCalculate(const int rates_total,
const int prev_calculated,
const datetime &time[],
const double &open[],
const double &high[],
const double &low[],
const double &close[],
const long &tick_volume[],
const long &volume[],
const int &spread[])
{
//---
ArraySetAsSeries(RSIBuffer,true);
ArraySetAsSeries(DeMarkerBuffer,true);
ArraySetAsSeries(SPBuffer,true);
ArraySetAsSeries(TmpDiverBuff,true);
ArraySetAsSeries(FastMABuffer,true);
ArraySetAsSeries(SlowMABuffer,true);
ArraySetAsSeries(RSI0,true);
ArraySetAsSeries(RSI1,true);
ArraySetAsSeries(RSI2,true);
ArraySetAsSeries(RSI3,true);
ArraySetAsSeries(DM0,true);
ArraySetAsSeries(DM1,true);
ArraySetAsSeries(DM2,true);
ArraySetAsSeries(DM3,true);
int rsi0=iRSI(_Symbol,0,RSI+SPStep*0,0);
int rsi1=iRSI(_Symbol,0,RSI+SPStep*1,0);
int rsi2=iRSI(_Symbol,0,RSI+SPStep*2,0);
int rsi3=iRSI(_Symbol,0,RSI+SPStep*3,0);
int dm0=iDeMarker(_Symbol,0,DeMarker+SPStep*0);
int dm1=iDeMarker(_Symbol,0,DeMarker+SPStep*1);
int dm2=iDeMarker(_Symbol,0,DeMarker+SPStep*2);
int dm3=iDeMarker(_Symbol,0,DeMarker+SPStep*3);
int fast=iMA(_Symbol,0,FastMA,0,0,0);
int slow=iMA(_Symbol,0,SlowMA,0,0,0);
CopyBuffer(rsi0,0,0,100,RSI0);
CopyBuffer(rsi1,0,0,100,RSI1);
CopyBuffer(rsi2,0,0,100,RSI2);
CopyBuffer(rsi3,0,0,100,RSI3);
CopyBuffer(dm0,0,0,100,DM0);
CopyBuffer(dm1,0,0,100,DM1);
CopyBuffer(dm2,0,0,100,DM2);
CopyBuffer(dm3,0,0,100,DM3);
//CopyBuffer(fast,0,0,100,FastMABuffer);
// CopyBuffer(slow,0,0,100,SlowMABuffer);
for(int i=0; i<100; i++)
{
RSIBuffer[i]=(RSI0[i]+RSI1[i]+RSI2[i]+RSI3[i])/400;
DeMarkerBuffer[i]=(DM0[i]+DM1[i]+DM2[i]+DM3[i])/4;
SPBuffer[i]=(RSIBuffer[i]+DeMarkerBuffer[i])/2;
TmpDiverBuff[i]=DeMarkerBuffer[i]-RSIBuffer[i];
}
ExponentialMAOnBuffer(rates_total,prev_calculated,0,FastMA,SPBuffer,FastMABuffer);
ExponentialMAOnBuffer(rates_total,prev_calculated,0,SlowMA,SPBuffer,SlowMABuffer);
//--- return value of prev_calculated for next call
return(rates_total);
}
//+------------------------------------------------------------------+
AM2