
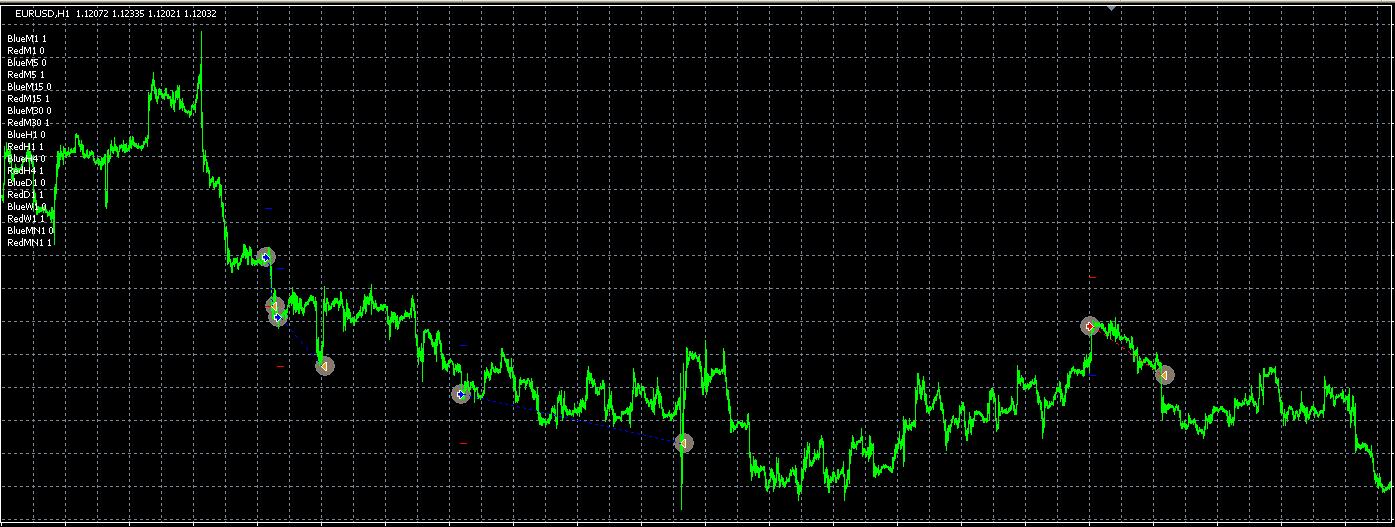
//+------------------------------------------------------------------+
//| Beton.mq4 |
//| Copyright 2015, AM2 |
//| http://www.forexsystems.biz |
//+------------------------------------------------------------------+
#property copyright "Copyright © 2015, AM2"
#property link "http://www.forexsystems.biz"
#property description "Simple expert advisor"
#define MAGICMA 20150127
//--- Inputs
extern double StopLoss = 500;
extern double TakeProfit = 500;
extern double Slip = 100;
extern double Lots = 0.1;
//----
extern int BuyM1 = 0;// signal = 1
extern int BuyM5 = 0;
extern int BuyM15 = 0;
extern int BuyM30 = 0;
extern int BuyH1 = 0;
extern int BuyH4 = 0;
extern int BuyD1 = 0;
extern int BuyW1 = 0;
extern int BuyMN1 = 0;
//----
extern int SellM1 = 0;// signal = 1
extern int SellM5 = 0;
extern int SellM15 = 0;
extern int SellM30 = 0;
extern int SellH1 = 0;
extern int SellH4 = 0;
extern int SellD1 = 0;
extern int SellW1 = 0;
extern int SellMN1 = 0;
//----
extern int K = 30;
extern double Kstop = 0.5;
extern int Kperiod = 150;
extern int PerADX = 7;
extern int CountBars = 350;
//----
int bm1,rm1,bm5,rm5,bm15,rm15,bm30,rm30,bh1,rh1,bh4,rh4,bd1,rd1,bw1,rw1,bmn1,rmn1;
//+------------------------------------------------------------------+
//| Check for open order conditions |
//+------------------------------------------------------------------+
void CheckForOpen()
{
int res;
//--- go trading only for first tiks of new bar
if(Volume[0]>1) return;
//--- sell conditions
if(rm1==SellM1 && rm5==SellM5 && rm15==SellM15 && rm30==SellM30 && rh1==SellH1 && rh4==SellH4 && rd1==SellD1 && rw1==SellW1 && rmn1==SellMN1)
{
res=OrderSend(Symbol(),OP_SELL,Lots,Bid,Slip,Bid+StopLoss*Point,Bid-TakeProfit*Point,"",MAGICMA,0,Red);
}
//--- buy conditions
if(bm1==BuyM1 && bm5==BuyM5 && bm15==BuyM15 && bm30==BuyM30 && bh1==BuyH1 && bh4==BuyH4 && bd1==BuyD1 && bw1==BuyW1 && bmn1==BuyMN1)
{
res=OrderSend(Symbol(),OP_BUY,Lots,Ask,Slip,Ask-StopLoss*Point,Ask+TakeProfit*Point,"",MAGICMA,0,Blue);
}
//---
}
//+------------------------------------------------------------------+
//| Check for close order conditions |
//+------------------------------------------------------------------+
void CheckForClose()
{
//--- go trading only for first tiks of new bar
if(Volume[0]>1) return;
//--- get Indicators
double AlBlue=iCustom(Symbol(),0,"AltrTrend",K,Kstop,Kperiod,PerADX,CountBars,1,1);
double AlRed=iCustom(Symbol(),0,"AltrTrend",K,Kstop,Kperiod,PerADX,CountBars,0,1);
//---
for(int i=0;i<OrdersTotal();i++)
{
if(OrderSelect(i,SELECT_BY_POS,MODE_TRADES)==false) break;
if(OrderMagicNumber()!=MAGICMA || OrderSymbol()!=Symbol()) continue;
//--- check order type
if(OrderType()==OP_BUY)
{
if(AlBlue>AlRed)
{
if(!OrderClose(OrderTicket(),OrderLots(),Bid,Slip,White))
Print("OrderClose error ",GetLastError());
}
break;
}
if(OrderType()==OP_SELL)
{
if(AlRed>AlBlue)
{
if(!OrderClose(OrderTicket(),OrderLots(),Ask,Slip,White))
Print("OrderClose error ",GetLastError());
}
break;
}
}
//---
}
//+------------------------------------------------------------------+
//| OnTick function |
//+------------------------------------------------------------------+
void OnTick()
{
double AlBlueM1=iCustom(Symbol(),PERIOD_M1,"AltrTrend",K,Kstop,Kperiod,PerADX,CountBars,0,1);
double AlRedM1=iCustom(Symbol(),PERIOD_M1,"AltrTrend",K,Kstop,Kperiod,PerADX,CountBars,1,1);
double AlBlueM5=iCustom(Symbol(),PERIOD_M5,"AltrTrend",K,Kstop,Kperiod,PerADX,CountBars,0,1);
double AlRedM5=iCustom(Symbol(),PERIOD_M5,"AltrTrend",K,Kstop,Kperiod,PerADX,CountBars,1,1);
double AlBlueM15=iCustom(Symbol(),PERIOD_M15,"AltrTrend",K,Kstop,Kperiod,PerADX,CountBars,0,1);
double AlRedM15=iCustom(Symbol(),PERIOD_M15,"AltrTrend",K,Kstop,Kperiod,PerADX,CountBars,1,1);
double AlBlueM30=iCustom(Symbol(),PERIOD_M30,"AltrTrend",K,Kstop,Kperiod,PerADX,CountBars,0,1);
double AlRedM30=iCustom(Symbol(),PERIOD_M30,"AltrTrend",K,Kstop,Kperiod,PerADX,CountBars,1,1);
double AlBlueH1=iCustom(Symbol(),PERIOD_H1,"AltrTrend",K,Kstop,Kperiod,PerADX,CountBars,0,1);
double AlRedH1=iCustom(Symbol(),PERIOD_H1,"AltrTrend",K,Kstop,Kperiod,PerADX,CountBars,1,1);
double AlBlueH4=iCustom(Symbol(),PERIOD_H4,"AltrTrend",K,Kstop,Kperiod,PerADX,CountBars,0,1);
double AlRedH4=iCustom(Symbol(),PERIOD_H4,"AltrTrend",K,Kstop,Kperiod,PerADX,CountBars,1,1);
double AlBlueD1=iCustom(Symbol(),PERIOD_D1,"AltrTrend",K,Kstop,Kperiod,PerADX,CountBars,0,1);
double AlRedD1=iCustom(Symbol(),PERIOD_D1,"AltrTrend",K,Kstop,Kperiod,PerADX,CountBars,1,1);
double AlBlueW1=iCustom(Symbol(),PERIOD_W1,"AltrTrend",K,Kstop,Kperiod,PerADX,CountBars,0,1);
double AlRedW1=iCustom(Symbol(),PERIOD_W1,"AltrTrend",K,Kstop,Kperiod,PerADX,CountBars,1,1);
double AlBlueMN1=iCustom(Symbol(),PERIOD_MN1,"AltrTrend",K,Kstop,Kperiod,PerADX,CountBars,0,1);
double AlRedMN1=iCustom(Symbol(),PERIOD_MN1,"AltrTrend",K,Kstop,Kperiod,PerADX,CountBars,1,1);
if(AlBlueM1>0) {bm1=1;rm1=0;}
if(AlRedM1>0) {rm1=1;bm1=0;}
if(AlBlueM5>0) {bm5=1;rm5=0;}
if(AlRedM5>0) {rm5=1;bm5=0;}
if(AlBlueM15>0) {bm15=1;rm15=0;}
if(AlRedM15>0) {rm15=1;bm15=0;}
if(AlBlueM30>0) {bm30=1;rm30=0;}
if(AlRedM30>0) {rm30=1;bm30=0;}
if(AlBlueH1>0) {bh1=1;rh1=0;}
if(AlRedH1>0) {rh1=1;bh1=0;}
if(AlBlueH4>0) {bh4=1;rh4=0;}
if(AlRedH4>0) {rh4=1;bh4=0;}
if(AlBlueD1>0) {bd1=1;rd1=0;}
if(AlRedD1>0) {rd1=1;bd1=0;}
if(AlBlueW1>0) {bw1=1;rw1=0;}
if(AlRedW1>0) {rw1=1;bw1=0;}
if(AlBlueMN1>0) {bmn1=1;rmn1=0;}
if(AlRedMN1>0) {rmn1=1;bmn1=0;}
Comment(
"\n BlueM1 ",bm1,
"\n RedM1 ",rm1,
"\n BlueM5 ",bm5,
"\n RedM5 ",rm5,
"\n BlueM15 ",bm15,
"\n RedM15 ",rm15,
"\n BlueM30 ",bm30,
"\n RedM30 ",rm30,
"\n BlueH1 ",bh1,
"\n RedH1 ",rh1,
"\n BlueH4 ",bh4,
"\n RedH4 ",rh4,
"\n BlueD1 ",bd1,
"\n RedD1 ",rd1,
"\n BlueW1 ",bw1,
"\n RedW1 ",rw1,
"\n BlueMN1 ",bmn1,
"\n RedMN1 ",rmn1);
if(OrdersTotal()<1) CheckForOpen();
//else CheckForClose();
//---
}
//+------------------------------------------------------------------+
//+------------------------------------------------------------------+
//| Beton.mq4 |
//| Copyright 2015, AM2 |
//| http://www.forexsystems.biz |
//+------------------------------------------------------------------+
#property copyright "Copyright © 2011, AM2"
#property link "http://www.forexsystems.biz"
#property description "Simple expert advisor"
#define MAGICMA 20150127
//--- Inputs
extern double StopLoss = 500;
extern double TakeProfit = 500;
extern double Slip = 100;
extern double Lots = 0.1;
//----
extern int M1 = 0;
extern int M5 = 0;
extern int M15 = 0;
extern int M30 = 0;
extern int H1 = 0;
extern int H4 = 0;
extern int D1 = 0;
extern int W1 = 0;
extern int MN = 0;
//----
extern int K = 30;
extern double Kstop = 0.5;
extern int Kperiod = 150;
extern int PerADX = 7;
extern int CountBars = 350;
//----
int bm1,rm1,bm5,rm5,bm15,rm15,bm30,rm30,bh1,rh1,bh4,rh4,bd1,rd1,bw1,rw1,bmn1,rmn1;
//+------------------------------------------------------------------+
//| Check for open order conditions |
//+------------------------------------------------------------------+
void CheckForOpen()
{
int res;
//--- go trading only for first tiks of new bar
if(Volume[0]>1) return;
//--- sell conditions
if(rm1>0 && rm5>0 && rm15>0 && rm30>0 && rh1>0 && rh4>0 && rd1>0)
{
res=OrderSend(Symbol(),OP_SELL,Lots,Bid,Slip,Bid+StopLoss*Point,Bid-TakeProfit*Point,"",MAGICMA,0,Red);
}
//--- buy conditions
if(bm1>0 && bm5>0 && bm15>0 && bm30>0 && bh1>0 && bh4>0 && bd1>0)
{
res=OrderSend(Symbol(),OP_BUY,Lots,Ask,Slip,Ask-StopLoss*Point,Ask+TakeProfit*Point,"",MAGICMA,0,Blue);
}
//---
}
//+------------------------------------------------------------------+
//| Check for close order conditions |
//+------------------------------------------------------------------+
void CheckForClose()
{
//--- go trading only for first tiks of new bar
if(Volume[0]>1) return;
//--- get Indicators
double AlBlue=iCustom(Symbol(),0,"AltrTrend",K,Kstop,Kperiod,PerADX,CountBars,1,1);
double AlRed=iCustom(Symbol(),0,"AltrTrend",K,Kstop,Kperiod,PerADX,CountBars,0,1);
//---
for(int i=0;i<OrdersTotal();i++)
{
if(OrderSelect(i,SELECT_BY_POS,MODE_TRADES)==false) break;
if(OrderMagicNumber()!=MAGICMA || OrderSymbol()!=Symbol()) continue;
//--- check order type
if(OrderType()==OP_BUY)
{
if(AlBlue>AlRed)
{
if(!OrderClose(OrderTicket(),OrderLots(),Bid,Slip,White))
Print("OrderClose error ",GetLastError());
}
break;
}
if(OrderType()==OP_SELL)
{
if(AlRed>AlBlue)
{
if(!OrderClose(OrderTicket(),OrderLots(),Ask,Slip,White))
Print("OrderClose error ",GetLastError());
}
break;
}
}
//---
}
//+------------------------------------------------------------------+
//| OnTick function |
//+------------------------------------------------------------------+
void OnTick()
{
double AlBlueM1=iCustom(Symbol(),PERIOD_M1,"AltrTrend",K,Kstop,Kperiod,PerADX,CountBars,0,1);
double AlRedM1=iCustom(Symbol(),PERIOD_M1,"AltrTrend",K,Kstop,Kperiod,PerADX,CountBars,1,1);
double AlBlueM5=iCustom(Symbol(),PERIOD_M5,"AltrTrend",K,Kstop,Kperiod,PerADX,CountBars,0,1);
double AlRedM5=iCustom(Symbol(),PERIOD_M5,"AltrTrend",K,Kstop,Kperiod,PerADX,CountBars,1,1);
double AlBlueM15=iCustom(Symbol(),PERIOD_M15,"AltrTrend",K,Kstop,Kperiod,PerADX,CountBars,0,1);
double AlRedM15=iCustom(Symbol(),PERIOD_M15,"AltrTrend",K,Kstop,Kperiod,PerADX,CountBars,1,1);
double AlBlueM30=iCustom(Symbol(),PERIOD_M30,"AltrTrend",K,Kstop,Kperiod,PerADX,CountBars,0,1);
double AlRedM30=iCustom(Symbol(),PERIOD_M30,"AltrTrend",K,Kstop,Kperiod,PerADX,CountBars,1,1);
double AlBlueH1=iCustom(Symbol(),PERIOD_H1,"AltrTrend",K,Kstop,Kperiod,PerADX,CountBars,0,1);
double AlRedH1=iCustom(Symbol(),PERIOD_H1,"AltrTrend",K,Kstop,Kperiod,PerADX,CountBars,1,1);
double AlBlueH4=iCustom(Symbol(),PERIOD_H4,"AltrTrend",K,Kstop,Kperiod,PerADX,CountBars,0,1);
double AlRedH4=iCustom(Symbol(),PERIOD_H4,"AltrTrend",K,Kstop,Kperiod,PerADX,CountBars,1,1);
double AlBlueD1=iCustom(Symbol(),PERIOD_D1,"AltrTrend",K,Kstop,Kperiod,PerADX,CountBars,0,1);
double AlRedD1=iCustom(Symbol(),PERIOD_D1,"AltrTrend",K,Kstop,Kperiod,PerADX,CountBars,1,1);
double AlBlueW1=iCustom(Symbol(),PERIOD_W1,"AltrTrend",K,Kstop,Kperiod,PerADX,CountBars,0,1);
double AlRedW1=iCustom(Symbol(),PERIOD_W1,"AltrTrend",K,Kstop,Kperiod,PerADX,CountBars,1,1);
double AlBlueMN1=iCustom(Symbol(),PERIOD_MN1,"AltrTrend",K,Kstop,Kperiod,PerADX,CountBars,0,1);
double AlRedMN1=iCustom(Symbol(),PERIOD_MN1,"AltrTrend",K,Kstop,Kperiod,PerADX,CountBars,1,1);
if(AlBlueM1>0) {bm1=1;rm1=0;}
if(AlRedM1>0) {rm1=1;bm1=0;}
if(AlBlueM5>0) {bm5=1;rm5=0;}
if(AlRedM5>0) {rm5=1;bm5=0;}
if(AlBlueM15>0) {bm15=1;rm15=0;}
if(AlRedM15>0) {rm15=1;bm15=0;}
if(AlBlueM30>0) {bm30=1;rm30=0;}
if(AlRedM30>0) {rm30=1;bm30=0;}
if(AlBlueH1>0) {bh1=1;rh1=0;}
if(AlRedH1>0) {rh1=1;bh1=0;}
if(AlBlueH4>0) {bh4=1;rh4=0;}
if(AlRedH4>0) {rh4=1;bh4=0;}
if(AlBlueD1>0) {bd1=1;rd1=0;}
if(AlRedD1>0) {rd1=1;bd1=0;}
if(AlBlueW1>0) {bw1=1;rw1=0;}
if(AlRedW1>0) {rw1=1;bw1=0;}
if(AlBlueMN1>0) {bmn1=1;rmn1=0;}
if(AlRedMN1>0) {rmn1=1;bmn1=0;}
Comment(
"\n BlueM1 ",bm1,
"\n RedM1 ",rm1,
"\n BlueM5 ",bm5,
"\n RedM5 ",rm5,
"\n BlueM15 ",bm15,
"\n RedM15 ",rm15,
"\n BlueM30 ",bm30,
"\n RedM30 ",rm30,
"\n BlueH1 ",bh1,
"\n RedH1 ",rh1,
"\n BlueH4 ",bh4,
"\n RedH4 ",rh4,
"\n BlueD1 ",bd1,
"\n RedD1 ",rd1,
"\n BlueW1 ",bw1,
"\n RedW1 ",rw1,
"\n BlueMN1 ",bmn1,
"\n RedMN1 ",rmn1);
if(OrdersTotal()<1) CheckForOpen();
//else CheckForClose();
//---
}
//+------------------------------------------------------------------+
//+------------------------------------------------------------------+
//| AMA2.mq4 |
//| Copyright 2015, AM2 |
//| http://www.forexsystems.biz |
//+------------------------------------------------------------------+
#property copyright "Copyright © 2015, AM2"
#property link "http://www.forexsystems.biz"
#property description "AMA simple expert advisor"
#define MAGICMA 20141020
//--- Inputs
extern double StopLoss = 500;
extern double TakeProfit = 500;
extern double Lots = 0.1;
extern int Shift = 1;
extern int Slip = 100;
//----
extern int PeriodAMA = 9;
extern int FastMA = 2;
extern int SlowMA = 30;
extern int G = 2;
extern int Dk = 2;
//-----
extern int PeriodAMA1 = 9;
extern int FastMA1 = 2;
extern int SlowMA1 = 30;
extern int G1 = 2;
extern int Dk1 = 2;
//----
int res;
//+------------------------------------------------------------------+
//| Check for open order conditions |
//+------------------------------------------------------------------+
void CheckForOpen()
{
//--- go trading only for first tiks of new bar
if(Volume[0]>1) return;
//--- get Moving Average
double AMABlue=iCustom(Symbol(),0,"AMA",PeriodAMA,FastMA,SlowMA,G,Dk,2,Shift);
double AMARed=iCustom(Symbol(),0,"AMA",PeriodAMA,FastMA,SlowMA,G,Dk,1,Shift);
double PrevAMABlue=iCustom(Symbol(),0,"AMA",PeriodAMA,FastMA,SlowMA,G,Dk,2,Shift+1);
double PrevAMARed=iCustom(Symbol(),0,"AMA",PeriodAMA,FastMA,SlowMA,G,Dk,1,Shift+1);
//+------------------------------------------------------------------+
//--- sell conditions
if(AMABlue>AMARed && PrevAMARed>PrevAMABlue)//AMA красная и на предыдущей свече синяя
{
res=OrderSend(Symbol(),OP_SELL,Lots,Bid,Slip,Bid+StopLoss*Point,Bid-TakeProfit*Point,"",MAGICMA,0,Red);
return;
}
//--- buy conditions
if(AMARed>AMABlue && PrevAMABlue>PrevAMARed)//AMA синяя и на предыдущей свече красная
{
res=OrderSend(Symbol(),OP_BUY,Lots,Ask,Slip,Ask-StopLoss*Point,Ask+TakeProfit*Point,"",MAGICMA,0,Blue);
return;
}
//---
}
//+------------------------------------------------------------------+
//| Check for close order conditions |
//+------------------------------------------------------------------+
void CheckForClose()
{
//--- go trading only for first tiks of new bar
if(Volume[0]>1) return;
//--- get Moving Average
double AMA1Blue=iCustom(Symbol(),0,"AMA",PeriodAMA1,FastMA1,SlowMA1,G1,Dk1,2,Shift);
double AMA1Red=iCustom(Symbol(),0,"AMA",PeriodAMA1,FastMA1,SlowMA1,G,Dk,1,Shift);
//---
for(int i=0;i<OrdersTotal();i++)
{
if(OrderSelect(i,SELECT_BY_POS,MODE_TRADES)==false) break;
if(OrderMagicNumber()!=MAGICMA || OrderSymbol()!=Symbol()) continue;
//--- check order type
if(OrderType()==OP_BUY)
{
if(AMA1Blue>AMA1Red && BuyProfit()>0)
{
CloseAllBuy();
}
break;
}
if(OrderType()==OP_SELL)
{
if(AMA1Red>AMA1Blue && SellProfit()>0)
{
CloseAllSell();
}
break;
}
}
//---
}
//+------------------------------------------------------------------+
void CloseAllSell()
{
bool cl,sel;
for (int i=OrdersTotal()-1;i>= 0;i--)
{
sel=OrderSelect(i,SELECT_BY_POS,MODE_TRADES);
if(OrderSymbol()==Symbol())
{
if(OrderSymbol()==Symbol() && OrderMagicNumber()==MAGICMA)
{
if(OrderType()==OP_SELL) cl=OrderClose(OrderTicket(),OrderLots(),Ask,Slip,Red);
}
Sleep(1000);
}
}
}
//+------------------------------------------------------------------+
void CloseAllBuy()
{
bool cl,sel;
for (int i=OrdersTotal()-1;i>= 0;i--)
{
sel=OrderSelect(i,SELECT_BY_POS,MODE_TRADES);
if(OrderSymbol()==Symbol())
{
if(OrderSymbol()==Symbol() && OrderMagicNumber()==MAGICMA)
{
if(OrderType()==OP_BUY) cl=OrderClose(OrderTicket(),OrderLots(),Bid,Slip,Blue);
}
Sleep(1000);
}
}
}
//+------------------------------------------------------------------+
double AllProfit()
{
double profit = 0;
for (int i=OrdersTotal()-1;i>=0;i--)
{
bool sel=OrderSelect(i,SELECT_BY_POS,MODE_TRADES);
if(OrderSymbol()!= Symbol() || OrderMagicNumber()!=MAGICMA) continue;
if(OrderSymbol()== Symbol() && OrderMagicNumber()==MAGICMA)
if(OrderType()==OP_BUY || OrderType()==OP_SELL) profit+=OrderProfit();
}
return (profit);
}
//+------------------------------------------------------------------+
double BuyProfit()
{
double profit = 0;
for (int i=OrdersTotal()-1;i>=0;i--)
{
bool sel=OrderSelect(i,SELECT_BY_POS,MODE_TRADES);
if(OrderSymbol()!= Symbol() || OrderMagicNumber()!=MAGICMA) continue;
if(OrderSymbol()== Symbol() && OrderMagicNumber()==MAGICMA)
if(OrderType()==OP_BUY) profit+=OrderProfit();
}
return (profit);
}
//+------------------------------------------------------------------+
double SellProfit()
{
double profit = 0;
for (int i=OrdersTotal()-1;i>=0;i--)
{
bool sel=OrderSelect(i,SELECT_BY_POS,MODE_TRADES);
if(OrderSymbol()!= Symbol() || OrderMagicNumber()!=MAGICMA) continue;
if(OrderSymbol()== Symbol() && OrderMagicNumber()==MAGICMA)
if(OrderType()==OP_SELL) profit+=OrderProfit();
}
return (profit);
}
//+------------------------------------------------------------------+
int CountBuyTrades()
{
int count;
for(int i=OrdersTotal()-1;i>=0;i--)
{
if(OrderSelect(i,SELECT_BY_POS,MODE_TRADES))
{
if(OrderSymbol()==Symbol() && OrderMagicNumber()==MAGICMA)
{
if(OrderType()==OP_BUY)
count++;
}
}
}
return(count);
}
//+------------------------------------------------------------------+
int CountSellTrades()
{
int count;
for(int i=OrdersTotal()-1;i>=0;i--)
{
if(OrderSelect(i,SELECT_BY_POS,MODE_TRADES))
{
if(OrderSymbol()==Symbol() && OrderMagicNumber()==MAGICMA)
{
if(OrderType()==OP_SELL)
count++;
}
}
}
return(count);
}
//+------------------------------------------------------------------+
//| OnTick function |
//+------------------------------------------------------------------+
void OnTick()
{
Comment("\n Открыто Buy ",CountBuyTrades(),
"\n Открыто Sell ",CountSellTrades(),
"\n Общий профит ",AllProfit(),
"\n Профит по Buy ",BuyProfit(),
"\n Профит по Sell ",SellProfit());
CheckForOpen();
CheckForClose();
//---
}
//+------------------------------------------------------------------+
//+------------------------------------------------------------------+
//| KuKlux.mq4 |
//| Copyright © 2015, AM2 |
//| http://www.forexsystems.biz |
//+------------------------------------------------------------------+
#property copyright "Copyright © 2015, AM2"
#property link "http://www.forexsystems.biz"
#property description "Forex expert advisor"
//--- Inputs
extern double StopLoss = 2; // на сколько делим волатильность для определения стоплосса ордера
extern double TakeProfit = 2; // на сколько делим волатильность для определения тейкпрофита ордера
extern double Delta = 3; // на сколько делим волатильность для определения расстояния от цены до ордера
extern int Expiration = 14; // время истечения ордера
extern int StartHour = 9; // время начала торговли
extern int Days = 1; // дни для расчета волатильности
extern double Lots = 0.1; // объем позиции
extern int MAGIC = 333; // магик
double price,h,l,vol,sl,tp;
//+------------------------------------------------------------------+
//| Check for open order conditions |
//+------------------------------------------------------------------+
void PutOrder()
{
int res,b,s;
datetime expiration = TimeCurrent()+3600*Expiration;
double bprice,sprice;
for(int i=OrdersTotal()-1;i>=0;i--)
{
if(OrderSelect(i,SELECT_BY_POS,MODE_TRADES))
{
if(OrderSymbol()==Symbol() && OrderMagicNumber()==MAGIC)
{
if(OrderType()==OP_BUYSTOP)b++;
if(OrderType()==OP_SELLSTOP)s++;
}
}
}
//--- buy
if(Hour()==StartHour && b<1)
{
bprice=fND(Ask+(vol/Delta));
sl=fND((vol/StopLoss));
tp=fND((vol/TakeProfit));
res=OrderSend(Symbol(),OP_BUYSTOP,Lots,fND(bprice),3,fND(bprice-sl),fND(bprice+tp),"",MAGIC,expiration,Blue);
}
//--- sell
if(Hour()==StartHour && s<1)
{
sprice=fND(Bid-(vol/Delta));
sl=fND((vol/StopLoss));
tp=fND((vol/TakeProfit));
res=OrderSend(Symbol(),OP_SELLSTOP,Lots,fND(sprice),3,fND(sprice+sl),fND(sprice-tp),"",MAGIC,expiration,Red);
}
}
//+------------------------------------------------------------------+
double Vol()
{
double Vol,H,L;
for(int i=1;i>=Days;i++)
{
H=iHigh(Symbol(),PERIOD_D1,Days);
L=iLow(Symbol(),PERIOD_D1,Days);
vol=(H-L)/Days;
}
return(Vol);
}
//+------------------------------------------------------------------+
void DelOrder()
{
bool del;
for(int i=OrdersTotal()-1; i>=0; i--)
{
if(OrderSelect(i,SELECT_BY_POS,MODE_TRADES)==false) break;
{
if(OrderMagicNumber()!=MAGIC || OrderSymbol()!=Symbol()) continue;
{
if (OrderSelect(i,SELECT_BY_POS,MODE_TRADES)==false) break;
if (OrderType()==OP_BUYSTOP) del=OrderDelete(OrderTicket());
if (OrderType()==OP_SELLSTOP) del=OrderDelete(OrderTicket());
}
}
}
}
//+------------------------------------------------------------------+
bool NewBar()
{
static datetime lastbar = 0;
datetime curbar = Time[0];
if(lastbar!=curbar)
{
lastbar=curbar;
return (true);
}
else
{
return(false);
}
}
//+------------------------------------------------------------------+
int CountTrades()
{
int count=0;
for(int i=OrdersTotal()-1;i>=0;i--)
{
if(OrderSelect(i,SELECT_BY_POS,MODE_TRADES))
{
if(OrderSymbol()==Symbol() && OrderMagicNumber()==MAGIC)
{
if(OrderType()==OP_BUY || OrderType()==OP_SELL)
count++;
}
}
}
return(count);
}
//+------------------------------------------------------------------+
double fND(double d,int n=-1)
{
if(n<0) return(NormalizeDouble(d, Digits));
return(NormalizeDouble(d, n));
}
//+------------------------------------------------------------------+
//| OnTick function |
//+------------------------------------------------------------------+
void OnTick()
{
h=iHigh(Symbol(),PERIOD_D1,Days);
l=iLow(Symbol(),PERIOD_D1,Days);
vol=h-l;
if(CountTrades()<1)PutOrder();
if(CountTrades()>0)DelOrder();
//---
Comment("\n Vol ",vol,
"\n High ",h,
"\n Low ",l,
"\n SL ",sl,
"\n TP ",tp);
}
//+------------------------------------------------------------------+
//+------------------------------------------------------------------+
//| AMA2.mq4 |
//| Copyright 2015, AM2 |
//| http://www.forexsystems.biz |
//+------------------------------------------------------------------+
#property copyright "Copyright © 2015, AM2"
#property link "http://www.forexsystems.biz"
#property description "AMA simple expert advisor"
#define MAGICMA 20141020
//--- Inputs
input double StopLoss = 500;
input double TakeProfit = 500;
input double Lots = 0.1;
//----
extern int FastMA = 2;
extern int SlowMA = 30;
extern int G = 2;
extern int Dk = 2;
//-----
extern int FastMA1 = 2;
extern int SlowMA1 = 30;
extern int G1 = 2;
extern int Dk1 = 2;
//----
int res,bp=0,sp=0;
//+------------------------------------------------------------------+
//| Check for open order conditions |
//+------------------------------------------------------------------+
void CheckForOpen()
{
//--- go trading only for first tiks of new bar
if(Volume[0]>1) return;
//--- get Moving Average
double AMABlue=iCustom(Symbol(),0,"AMA",FastMA,SlowMA,G,Dk,2,1);
double AMARed=iCustom(Symbol(),0,"AMA",FastMA,SlowMA,G,Dk,1,1);
//double AMA1Blue=iCustom(Symbol(),0,"AMA",FastMA1,SlowMA1,G1,Dk1,0,1);
//double AMA1Red=iCustom(Symbol(),0,"AMA",FastMA1,SlowMA1,G,Dk,1,1);
//+------------------------------------------------------------------+
for(int i=OrdersTotal()-1;i>=0;i--)
{
if(OrderSelect(i, SELECT_BY_POS))
{
if (OrderSymbol()!=Symbol() || OrderMagicNumber()!=MAGICMA) continue;
if (OrderType()==OP_BUY) bp++;
if (OrderType()==OP_SELL) sp++;
}
}
//+------------------------------------------------------------------+
//--- sell conditions
if(AMABlue>AMARed && sp<1)
{
res=OrderSend(Symbol(),OP_SELL,Lots,Bid,3,Bid+StopLoss*Point,Bid-TakeProfit*Point,"",MAGICMA,0,Red);
return;
}
//--- buy conditions
if(AMARed>AMABlue && bp<1)
{
res=OrderSend(Symbol(),OP_BUY,Lots,Ask,3,Ask-StopLoss*Point,Ask+TakeProfit*Point,"",MAGICMA,0,Blue);
return;
}
//---
}
//+------------------------------------------------------------------+
//| Check for close order conditions |
//+------------------------------------------------------------------+
void CheckForClose()
{
//--- go trading only for first tiks of new bar
if(Volume[0]>1) return;
//--- get Moving Average
double AMABlue=iCustom(Symbol(),0,"AMA",FastMA,SlowMA,G,Dk,2,1);
double AMARed=iCustom(Symbol(),0,"AMA",FastMA,SlowMA,G,Dk,1,1);
//double AMA1Blue=iCustom(Symbol(),0,"AMA",FastMA1,SlowMA1,G1,Dk1,0,1);
//double AMA1Red=iCustom(Symbol(),0,"AMA",FastMA1,SlowMA1,G,Dk,1,1);
//---
for(int i=0;i<OrdersTotal();i++)
{
if(OrderSelect(i,SELECT_BY_POS,MODE_TRADES)==false) break;
if(OrderMagicNumber()!=MAGICMA || OrderSymbol()!=Symbol()) continue;
//--- check order type
if(OrderType()==OP_BUY)
{
if(AMABlue>AMARed)
{
if(!OrderClose(OrderTicket(),OrderLots(),Bid,3,White))
Print("OrderClose error ",GetLastError());
}
break;
}
if(OrderType()==OP_SELL)
{
if(AMARed>AMABlue)
{
if(!OrderClose(OrderTicket(),OrderLots(),Ask,3,White))
Print("OrderClose error ",GetLastError());
}
break;
}
}
//---
}
//+------------------------------------------------------------------+
double AllProfit()
{
double profit = 0;
for (int i=OrdersTotal()-1;i>=0;i--)
{
bool sel=OrderSelect(i,SELECT_BY_POS,MODE_TRADES);
if(OrderSymbol()!= Symbol() || OrderMagicNumber()!=MAGICMA) continue;
if(OrderSymbol()== Symbol() && OrderMagicNumber()==MAGICMA)
if(OrderType()==OP_BUY || OrderType()==OP_SELL) profit+=OrderProfit();
}
return (profit);
}
//+------------------------------------------------------------------+
double BuyProfit()
{
double profit = 0;
for (int i=OrdersTotal()-1;i>=0;i--)
{
bool sel=OrderSelect(i,SELECT_BY_POS,MODE_TRADES);
if(OrderSymbol()!= Symbol() || OrderMagicNumber()!=MAGICMA) continue;
if(OrderSymbol()== Symbol() && OrderMagicNumber()==MAGICMA)
if(OrderType()==OP_BUY) profit+=OrderProfit();
}
return (profit);
}
//+------------------------------------------------------------------+
double SellProfit()
{
double profit = 0;
for (int i=OrdersTotal()-1;i>=0;i--)
{
bool sel=OrderSelect(i,SELECT_BY_POS,MODE_TRADES);
if(OrderSymbol()!= Symbol() || OrderMagicNumber()!=MAGICMA) continue;
if(OrderSymbol()== Symbol() && OrderMagicNumber()==MAGICMA)
if(OrderType()==OP_SELL) profit+=OrderProfit();
}
return (profit);
}
//+------------------------------------------------------------------+
//| OnTick function |
//+------------------------------------------------------------------+
void OnTick()
{
//--- check for history and trading
if(Bars<100 || IsTradeAllowed()==false)
return;
//--- calculate open orders by current symbol
CheckForOpen();
CheckForClose();
//---
//+------------------------------------------------------------------+
for(int i=OrdersTotal()-1;i>=0;i--)
{
if(OrderSelect(i, SELECT_BY_POS))
{
if (OrderSymbol()!=Symbol() || OrderMagicNumber()!=MAGICMA) continue;
if (OrderType()==OP_BUY) bp++;
if (OrderType()==OP_SELL) sp++;
}
}
if(OrdersTotal()<1)bp=0;sp=0;
Comment("\n Открыто Buy ",bp,
"\n Открыто Sell ",sp,
"\n Общий профит ",AllProfit(),
"\n Профит по Buy ",BuyProfit(),
"\n Профит по Sell ",SellProfit());
}
//+------------------------------------------------------------------+
f0_14("MST1Line", Ai_12, NormalizeDouble(Gda_380[Ai_0], Gi_544), Ai_4, NormalizeDouble(Gda_380[Ai_0], Gi_544), 1, STYLE_DASH, ST1_Color);
void f0_14(string As_0, int A_datetime_8, double A_price_12, int A_datetime_20, double A_price_24, double A_width_32, double A_style_40, color A_color_48)
//+------------------------------------------------------------------+
//| Sluggish.mq4 |
//| Copyright 2014, AM2 |
//| http://www.forexsystems.biz |
//+------------------------------------------------------------------+
#property copyright "Copyright © 2011, AM2"
#property link "http://www.forexsystems.biz"
#property description "Simple expert advisor"
#define MAGIC 20141020
//--- Inputs
extern int StopLoss = 1000; // Стоплосс ордера
extern int TakeProfit = 1000; // Тейкпрофит ордера
extern int TrailingStop = 1000; // Трал ордера
extern int Slip = 3; // Проскальзывание цены
extern int Shift = 1; // Индекс получаемого значения из индикаторного буфера (сдвиг относительно текущего бара на указанное количество периодов назад).
extern int ClosePos = 0; // 0-закрытие по ТП, 1-по сигналу индикатора
extern int Risk = 0; // Риск на сделку в % от депо
extern double Lots = 0.1; // Лот
//----
extern int BBPer = 20; // Период индикатора
extern double BBDev = 1; // Отклонение индикатора
//----
//+------------------------------------------------------------------+
//| Check for open order conditions |
//+------------------------------------------------------------------+
void CheckForOpen()
{
int res,b,s;
double tp,sl;
double BBBuy=iCustom(Symbol(),0,"3Bollinger_Bands_Stop_v2",BBPer,BBDev,2,Shift);
double BBSell=iCustom(Symbol(),0,"3Bollinger_Bands_Stop_v2",BBPer,BBDev,3,Shift);
for(int i=OrdersTotal()-1;i>=0;i--)
{
if(OrderSelect(i,SELECT_BY_POS,MODE_TRADES))
{
if(OrderSymbol()==Symbol() && OrderMagicNumber()==MAGIC)
{
if(OrderType()==OP_BUY)b++;
if(OrderType()==OP_SELL)s++;
}
}
}
//--- sell conditions
if(BBSell>0 && s<1)
{
sl=Bid+StopLoss*Point;
tp=Bid-TakeProfit*Point;
if(StopLoss==0)sl=0;
if(TakeProfit==0)tp=0;
res=OrderSend(Symbol(),OP_SELL,fLots(),Bid,Slip,sl,tp,"",MAGIC,0,Red);
}
//--- buy conditions
if(BBBuy>0 && b<1)
{
sl=Ask-StopLoss*Point;
tp=Ask+TakeProfit*Point;
if(StopLoss==0)sl=0;
if(TakeProfit==0)tp=0;
res=OrderSend(Symbol(),OP_BUY,fLots(),Ask,Slip,sl,tp,"",MAGIC,0,Blue);
}
Comment("\nBuy ",BBBuy, "\nSell ",BBSell);
//---
}
//+------------------------------------------------------------------+
//| Check for close order conditions |
//+------------------------------------------------------------------+
void CheckForClose()
{
double BBBuy=iCustom(Symbol(),0,"3Bollinger_Bands_Stop_v2",BBPer,BBDev,2,Shift);
double BBSell=iCustom(Symbol(),0,"3Bollinger_Bands_Stop_v2",BBPer,BBDev,3,Shift);
//---
for(int i=0;i<OrdersTotal();i++)
{
if(OrderSelect(i,SELECT_BY_POS,MODE_TRADES)==false) break;
if(OrderMagicNumber()!=MAGIC || OrderSymbol()!=Symbol()) continue;
//--- check order type
if(OrderType()==OP_BUY)
{
if(BBSell>0)
{
if(!OrderClose(OrderTicket(),OrderLots(),Bid,Slip,White))
Print("OrderClose error ",GetLastError());
}
break;
}
if(OrderType()==OP_SELL)
{
if(BBBuy>0)
{
if(!OrderClose(OrderTicket(),OrderLots(),Ask,Slip,White))
Print("OrderClose error ",GetLastError());
}
break;
}
}
//---
}
//+------------------------------------------------------------------+
double fLots()
{
double lot=Lots;
double lot_min=MarketInfo( Symbol(),MODE_MINLOT);
double lot_max=MarketInfo( Symbol(),MODE_MAXLOT);
if (Risk>0)
{
lot=fND(AccountBalance()*Risk/100000,2);
}
if (lot<lot_min) lot=lot_min;
if (lot>lot_max) lot=lot_max;
return(lot);
}
//+------------------------------------------------------------------+
double fND(double d,int n=-1)
{
if(n<0) return(NormalizeDouble(d, Digits));
return(NormalizeDouble(d, n));
}
//--------------------------------------------------------------------
void Trailing()
{
for(int i=0; i<OrdersTotal(); i++)
{
if(OrderSelect(i,SELECT_BY_POS,MODE_TRADES))
if(OrderSymbol()==Symbol() || OrderMagicNumber()==MAGIC)
if(OrderType()==OP_BUY)
{
if(TrailingStop>0)
{
if(Bid-OrderOpenPrice()>TrailingStop*Point)
{
if(OrderStopLoss()<Bid-TrailingStop*Point)
{
bool mod=OrderModify(OrderTicket(),OrderOpenPrice(),Bid-TrailingStop*Point,OrderTakeProfit(),0,Blue);
}
}
}
}
if(OrderType()==OP_SELL)
{
if(TrailingStop>0)
{
if((OrderOpenPrice()-Ask)>TrailingStop*Point)
{
if((OrderStopLoss()>(Ask+TrailingStop*Point)) || (OrderStopLoss()==0))
{
mod=OrderModify(OrderTicket(),OrderOpenPrice(),Ask+TrailingStop*Point,OrderTakeProfit(),0,Red);
}
}
}
}
}
}
//+------------------------------------------------------------------+
//| OnTick function |
//+------------------------------------------------------------------+
void OnTick()
{
//--- check for history and trading
if(Bars<100 || IsTradeAllowed()==false)
return;
//--- calculate open orders by current symbol
CheckForOpen();
if(ClosePos>0) CheckForClose();
if(TrailingStop!=0) Trailing();
//---
}
//+------------------------------------------------------------------+
2006.06.21 11:14:39;1.2634
2006.06.21 11:14:48;1.2633
2006.06.21 11:14:50;1.2634
2006.06.21 11:14:52;1.2633
--------------------------Connection lost
2006.06.21 11:18:13;1.2634
2006.06.21 11:18:23;1.2633
2006.06.21 11:18:29;1.2634
2006.06.21 11:18:37;1.2633
2006.06.21 04:06:15;1.2618
2006.06.21 04:06:36;1.2617
2006.06.21 04:06:38;1.2618
2006.06.21 04:06:41;1.2617
2006.06.21 04:06:42;1.2618
--------------------------Expert was stoped
2006.06.21 08:18:22;1.2618
2006.06.21 08:18:27;1.2619
2006.06.21 08:18:31;1.2618
2006.06.21 08:18:31;1.2619
2006.06.21 08:18:32;1.2621
AM2