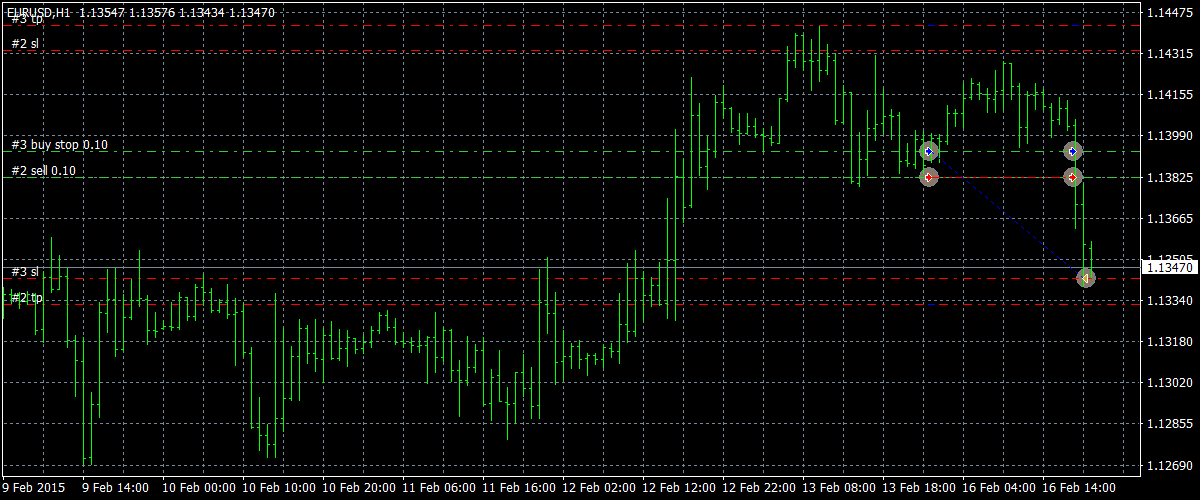
//+------------------------------------------------------------------+
//| MACDDiv.mq4 |
//| Copyright 2015, AM2 |
//| http://www.forexsystems.biz |
//+------------------------------------------------------------------+
#property copyright "Copyright © 2015, AM2"
#property link "http://www.forexsystems.biz"
#property description "Simple expert advisor"
//--- Inputs
extern int StopLoss = 500;
extern int TakeProfit = 500;
extern int BULevel = 0; // уровень БУ
extern int BUPoint = 30; // пункты БУ
extern int TrailingStop = 0; // трал
extern int TrailingStep = 20; // шаг трала
extern int Slip = 30;
extern int Magic = 123;
extern double Lots = 0.1;
//----
extern int SnakePeriod = 16;
extern int InpDepth = 12;
extern int InpDeviation = 5;
extern int InpBackstep = 3;
int t=0;
//+------------------------------------------------------------------+
//| Expert initialization function |
//+------------------------------------------------------------------+
int OnInit()
{
//---
//---
return(INIT_SUCCEEDED);
}
//+------------------------------------------------------------------+
//| Expert deinitialization function |
//+------------------------------------------------------------------+
void OnDeinit(const int reason)
{
//---
}
//+------------------------------------------------------------------+
//| Check for open order conditions |
//+------------------------------------------------------------------+
void OpenPos()
{
int r=0;
double sl=0,tp=0;
//--- get Ind
double UP=iCustom(Symbol(),0,"FX5 MACD_Divergence V1.1",0,2);
double DN=iCustom(Symbol(),0,"FX5 MACD_Divergence V1.1",1,2);
//--- sell conditions
if(DN!=2147483647)
{
if(StopLoss>0) sl=NormalizeDouble(Bid+StopLoss*Point,Digits);
if(TakeProfit>0) tp=NormalizeDouble(Bid-TakeProfit*Point,Digits);
r=OrderSend(Symbol(),OP_SELL,Lots,NormalizeDouble(Bid,Digits),Slip,sl,tp,"",Magic,0,Red);
}
//--- buy conditions
if(UP!=2147483647)
{
if(StopLoss>0) sl=NormalizeDouble(Ask-StopLoss*Point,Digits);
if(TakeProfit>0) tp=NormalizeDouble(Ask+TakeProfit*Point,Digits);
r=OrderSend(Symbol(),OP_BUY,Lots,NormalizeDouble(Ask,Digits),Slip,sl,tp,"",Magic,0,Blue);
}
//---
}
//+------------------------------------------------------------------+
//| Check for close order conditions |
//+------------------------------------------------------------------+
void ClosePos()
{
//--- get Ind
double UP=iCustom(Symbol(),0,"FX5 MACD_Divergence V1.1",0,2);
double DN=iCustom(Symbol(),0,"FX5 MACD_Divergence V1.1",1,2);
//---
for(int i=OrdersTotal()-1;i>=0;i--)
{
if(OrderSelect(i,SELECT_BY_POS,MODE_TRADES))
{
if(OrderMagicNumber()==Magic || OrderSymbol()==Symbol())
{
//--- check order type
if(OrderType()==OP_BUY)
{
if(DN!=2147483647)
{
CloseAll();
}
}
if(OrderType()==OP_SELL)
{
if(UP!=2147483647)
{
CloseAll();
}
}
}
}
}
return;
}
//+------------------------------------------------------------------+
//| |
//+------------------------------------------------------------------+
void CloseAll()
{
bool del;
for(int i=OrdersTotal()-1;i>=0;i--)
{
if(OrderSelect(i,SELECT_BY_POS,MODE_TRADES))
{
if(OrderSymbol()==Symbol() && OrderMagicNumber()==Magic)
{
if(OrderType()==OP_BUY) del=OrderClose(OrderTicket(),OrderLots(),Bid,Slip,White);
if(OrderType()==OP_SELL) del=OrderClose(OrderTicket(),OrderLots(),Bid,Slip,White);
}
}
}
return;
}
//+------------------------------------------------------------------+
int CountTrades()
{
int count=0;
for(int i=OrdersTotal()-1;i>=0;i--)
{
if(OrderSelect(i,SELECT_BY_POS,MODE_TRADES))
{
if(OrderSymbol()==Symbol() && OrderMagicNumber()==Magic)
{
if(OrderType()==OP_BUY || OrderType()==OP_SELL) count++;
}
}
}
return(count);
}
//+------------------------------------------------------------------+
//| |
//+------------------------------------------------------------------+
void Trailing()
{
bool mod;
for(int i=OrdersTotal()-1;i>=0;i--)
{
if(OrderSelect(i,SELECT_BY_POS,MODE_TRADES))
{
if(OrderType()==OP_BUY)
{
if(Bid-OrderOpenPrice()>TrailingStop*Point)
{
if(OrderStopLoss()<Bid-(TrailingStop+TrailingStep-1)*Point)
{
mod=OrderModify(OrderTicket(),OrderOpenPrice(),Bid-TrailingStop*Point,OrderTakeProfit(),0,Yellow);
return;
}
}
}
if(OrderType()==OP_SELL)
{
if((OrderOpenPrice()-Ask)>TrailingStop*Point)
{
if(OrderStopLoss()>Ask+(TrailingStop+TrailingStep-1)*Point || OrderStopLoss()==0)
{
mod=OrderModify(OrderTicket(),OrderOpenPrice(),Ask+TrailingStop*Point,OrderTakeProfit(),0,Yellow);
return;
}
}
}
}
}
}
//+------------------------------------------------------------------+
//| |
//+------------------------------------------------------------------+
void BU()
{
bool m;
for(int i=OrdersTotal()-1;i>=0;i--)
{
if(OrderSelect(i,SELECT_BY_POS,MODE_TRADES))
{
if(OrderSymbol()==Symbol() && OrderMagicNumber()==Magic)
{
if(OrderType()==OP_BUY)
{
if(OrderOpenPrice()<=(Bid-(BULevel+BUPoint)*Point) && OrderOpenPrice()>OrderStopLoss())
{
m=OrderModify(OrderTicket(),OrderOpenPrice(),OrderOpenPrice()+BUPoint*Point,OrderTakeProfit(),0,Yellow);
return;
}
}
if(OrderType()==OP_SELL)
{
if(OrderOpenPrice()>=(Ask+(BULevel+BUPoint)*Point) && (OrderOpenPrice()<OrderStopLoss() || OrderStopLoss()==0))
{
m=OrderModify(OrderTicket(),OrderOpenPrice(),OrderOpenPrice()-BUPoint*Point,OrderTakeProfit(),0,Yellow);
return;
}
}
}
}
}
}
//+------------------------------------------------------------------+
//| OnTick function |
//+------------------------------------------------------------------+
void OnTick()
{
double UP=iCustom(Symbol(),0,"FX5 MACD_Divergence V1.1",0,2);
double DN=iCustom(Symbol(),0,"FX5 MACD_Divergence V1.1",1,2);
if(BULevel>0) BU();
if(TrailingStop>0) Trailing();
if(t!=Time[0])
{
OpenPos();
t=Time[0];
}
//ClosePos();
Comment("\n UP: ",UP,
"\n DN: ",DN);
}
//+------------------------------------------------------------------+
Я про ваш будущий код на следующей недели по моему ТЗ)
Но попробовать не получилось попал к компу в момент закрытия рынка
Прошу ещё настройку для спреда добавить для большей точности результатов
AM2