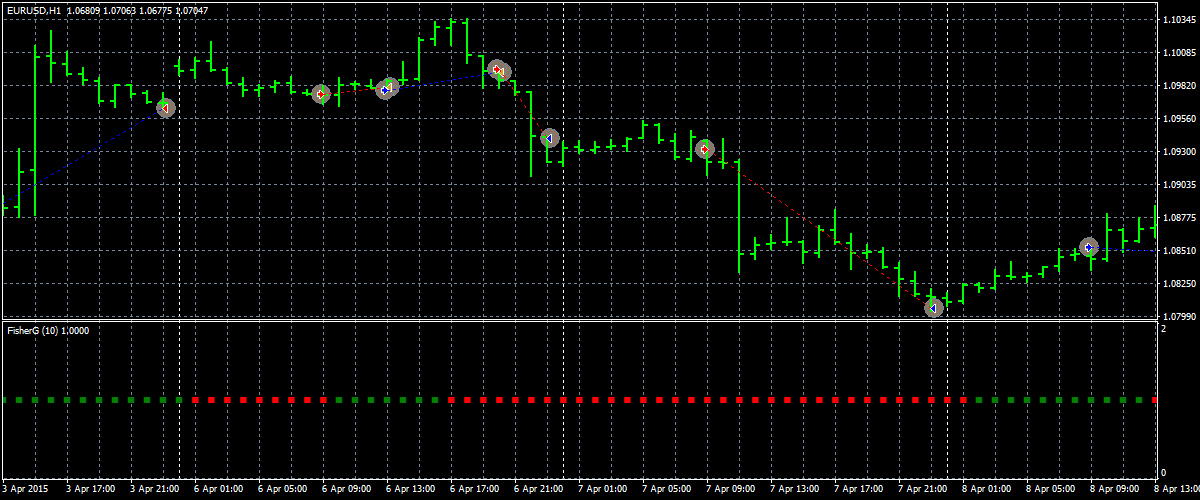
//+------------------------------------------------------------------+
//| Limit.mq4 |
//| Copyright 2015, AM2 |
//| http://www.forexsystems.biz |
//+------------------------------------------------------------------+
#property copyright "Copyright © 2015, AM2"
#property link "http://www.forexsystems.biz"
#property description "Simple expert advisor"
//--- Inputs
extern double Lots = 0.1; // лот
extern int StopLoss = 500; // лось
extern int TakeProfit = 500; // язь
extern int TrailingStop = 300; // трал
extern int StopLimit = 0; // 0-стоп 1-лимит
extern int Delta = 100; // отступ
extern int Slip = 30; // реквот
extern int StartHour = 9; // час начала торговли
extern int StartMin = 30; // минута начала торговли
extern int CloseHour = 3; // через сколько часов закрываем ордер
extern int Magic = 123; // магик
//+------------------------------------------------------------------+
//| Expert initialization function |
//+------------------------------------------------------------------+
int OnInit()
{
//---
Comment("");
//---
return(INIT_SUCCEEDED);
}
//+------------------------------------------------------------------+
//| Expert deinitialization function |
//+------------------------------------------------------------------+
void OnDeinit(const int reason)
{
Comment("");
}
//+------------------------------------------------------------------+
//| Check for open order conditions |
//+------------------------------------------------------------------+
void PutOrder(int type,double price)
{
int r=0;
double sl=0,tp=0;
if(type==1 || type==3 || type==5)
{
if(StopLoss>0) sl=NormalizeDouble(price+StopLoss*Point,Digits);
if(TakeProfit>0) tp=NormalizeDouble(price-TakeProfit*Point,Digits);
}
if(type==0 || type==2 || type==4)
{
if(StopLoss>0) sl=NormalizeDouble(price-StopLoss*Point,Digits);
if(TakeProfit>0) tp=NormalizeDouble(price+TakeProfit*Point,Digits);
}
r=OrderSend(NULL,type,Lots,NormalizeDouble(price,Digits),Slip,sl,tp,"",Magic,0,Green);
return;
//---
}
//+------------------------------------------------------------------+
//| |
//+------------------------------------------------------------------+
int CountTrades()
{
int count=0;
for(int i=OrdersTotal()-1;i>=0;i--)
{
if(OrderSelect(i,SELECT_BY_POS,MODE_TRADES))
{
if(OrderSymbol()==Symbol() && OrderMagicNumber()==Magic)
{
if(OrderType()==OP_BUY || OrderType()==OP_SELL) count++;
}
}
}
return(count);
}
//+------------------------------------------------------------------+
//| |
//+------------------------------------------------------------------+
int CountOrders(int type)
{
int count=0;
for(int i=OrdersTotal()-1;i>=0;i--)
{
if(OrderSelect(i,SELECT_BY_POS,MODE_TRADES))
{
if(OrderSymbol()==Symbol() && OrderMagicNumber()==Magic)
{
if(OrderType()==type) count++;
}
}
}
return(count);
}
//+------------------------------------------------------------------+
//| |
//+------------------------------------------------------------------+
void DelOrder()
{
bool del;
for(int i=OrdersTotal()-1;i>=0;i--)
{
if(OrderSelect(i,SELECT_BY_POS,MODE_TRADES))
{
if(OrderSymbol()==Symbol() && OrderMagicNumber()==Magic)
{
if(OrderType()>1) del=OrderDelete(OrderTicket());
}
}
}
return;
}
//+------------------------------------------------------------------+
//| |
//+------------------------------------------------------------------+
datetime OpenTime()
{
datetime t=0;
for(int i=OrdersTotal()-1;i>=0;i--)
{
if(OrderSelect(i,SELECT_BY_POS,MODE_TRADES))
{
if(OrderSymbol()==Symbol() && OrderMagicNumber()==Magic)
{
if(OrderType()<2) t=OrderOpenTime();
}
}
}
return(t);
}
//+------------------------------------------------------------------+
//| |
//+------------------------------------------------------------------+
int CloseTime()
{
int t=0;
if(OrderSelect(OrdersHistoryTotal()-1,SELECT_BY_POS,MODE_HISTORY)==true)
t=TimeDay(OrderCloseTime());
return(t);
}
//+------------------------------------------------------------------+
//| Check for close order conditions |
//+------------------------------------------------------------------+
void ClosePos()
{
bool c;
for(int i=OrdersTotal()-1;i>=0;i--)
{
if(OrderSelect(i,SELECT_BY_POS,MODE_TRADES))
{
if(OrderMagicNumber()==Magic || OrderSymbol()==Symbol())
{
if(OrderType()==OP_BUY)
{
c=OrderClose(OrderTicket(),OrderLots(),Bid,Slip,White);
break;
}
if(OrderType()==OP_SELL)
{
c=OrderClose(OrderTicket(),OrderLots(),Ask,Slip,White);
break;
}
}
}
}
}
//+------------------------------------------------------------------+
//| |
//+------------------------------------------------------------------+
void Trailing()
{
bool mod;
for(int i=0; i<OrdersTotal(); i++)
{
if(OrderSelect(i,SELECT_BY_POS,MODE_TRADES))
{
if(OrderSymbol()==Symbol() || OrderMagicNumber()==Magic)
{
if(OrderType()==OP_BUY)
{
if(TrailingStop>0)
{
if(Bid-OrderOpenPrice()>TrailingStop*Point)
{
if(OrderStopLoss()<Bid-TrailingStop*Point)
{
mod=OrderModify(OrderTicket(),OrderOpenPrice(),Bid-TrailingStop*Point,OrderTakeProfit(),0,Yellow);
return;
}
}
}
}
if(OrderType()==OP_SELL)
{
if(TrailingStop>0)
{
if((OrderOpenPrice()-Ask)>TrailingStop*Point)
{
if((OrderStopLoss()>(Ask+TrailingStop*Point)) || (OrderStopLoss()==0))
{
mod=OrderModify(OrderTicket(),OrderOpenPrice(),Ask+TrailingStop*Point,OrderTakeProfit(),0,Yellow);
return;
}
}
}
}
}
}
}
}
//+------------------------------------------------------------------+
//| OnTick function |
//+------------------------------------------------------------------+
void OnTick()
{
/*
Тип операции текущего выбранного ордера. Mожет быть одной из следующих величин:
0 - OP_BUY - ордер на покупку,
1 - OP_SELL - ордер на продажу,
2 - OP_BUYLIMIT - отложенный ордер на покупку по достижении заданного уровня, текущая цена выше уровня,
3 - OP_SELLLIMIT - отложенный ордер на продажу по достижении заданного уровня, текущая цена ниже уровня,
4 - OP_BUYSTOP - отложенный ордер на покупку по достижении заданного уровня, текущая цена ниже уровня,
5 - OP_SELLSTOP - отложенный ордер на продажу по достижении заданного уровня, текущая цена выше уровня.
*/
if(Hour()>=StartHour && Minute()>=StartMin)
{
if(CountTrades()<1 /*&& Day()!=CloseTime()*/)
{
if(StopLimit==0)
{
if(CountOrders(5)<1)PutOrder(5,Bid-Delta*Point);
if(CountOrders(4)<1)PutOrder(4,Bid+Delta*Point);
}
if(StopLimit==1)
{
if(CountOrders(2)<1)PutOrder(2,Bid-Delta*Point);
if(CountOrders(3)<1)PutOrder(3,Bid+Delta*Point);
}
}
}
if(CountTrades()>0) DelOrder();
if(CountTrades()>0 && TimeCurrent()-OpenTime()>CloseHour*3600) ClosePos();
if(TrailingStop>0) Trailing();
}
//+------------------------------------------------------------------+
//+------------------------------------------------------------------+
//| Bars.mq4 |
//| Copyright 2016, AM2 |
//| http://www.forexsystems.biz |
//+------------------------------------------------------------------+
#property copyright "Copyright 2016, AM2"
#property link "http://www.forexsystems.biz"
#property version "1.00"
#property strict
#property indicator_chart_window
input int bars = 150; //баров для расчета
input int width = 1; //толщина линии
input string Shift = "04:00:00"; //сдвиг линий по времени
input string TimeShift = "01:05"; //сдвиг первой линии по времени
input string Name = "VLine";
input color clr = clrAliceBlue;
datetime tm=0;
//+------------------------------------------------------------------+
//| Custom indicator initialization function |
//+------------------------------------------------------------------+
int OnInit()
{
//--- indicator buffers mapping
ObjectsDeleteAll(0,OBJ_VLINE);
Comment("");
//---
return(INIT_SUCCEEDED);
}
//+------------------------------------------------------------------+
//| Custom indicator deinitialization function |
//+------------------------------------------------------------------+
void OnDeinit(const int reason)
{
ObjectsDeleteAll(0,OBJ_VLINE);
Comment("");
}
//+------------------------------------------------------------------+
//| |
//+------------------------------------------------------------------+
void PutVLine(string name,datetime t)
{
ObjectCreate(name,OBJ_VLINE,0,t,0);
ObjectSet(name,OBJPROP_COLOR,clr);
ObjectSet(name,OBJPROP_STYLE,STYLE_SOLID);
ObjectSet(name,OBJPROP_WIDTH,width);
}
//+------------------------------------------------------------------+
//| Custom indicator iteration function |
//+------------------------------------------------------------------+
int OnCalculate(const int rates_total,
const int prev_calculated,
const datetime &time[],
const double &open[],
const double &high[],
const double &low[],
const double &close[],
const long &tick_volume[],
const long &volume[],
const int &spread[])
{
//---
int shift=iBarShift(NULL,0,StringToTime(TimeShift));
if(tm!=time[0])
{
ObjectsDeleteAll(0,OBJ_VLINE);
for(int i=shift;i<=bars;i++)
{
PutVLine(Name+TimeToStr(time[i]),time[i]);
i=i+iBarShift(NULL,0,StringToTime(Shift));
}
tm=time[0];
}
Comment("\n Shift:",shift);
//--- return value of prev_calculated for next call
return(rates_total);
}
//+------------------------------------------------------------------+
Какой код сделать чтобы
input int bar = 4; //расстояние между линиями
было 4.1 например? Вводил double но не сдвигается.
//+------------------------------------------------------------------+
//| Div.mq4 |
//| Copyright 2016, AM2 |
//| http://www.forexsystems.biz |
//+------------------------------------------------------------------+
#property copyright "Copyright © 2016, AM2"
#property link "http://www.forexsystems.biz"
#property description "simple expert advisor"
//--- Inputs
extern int StopLoss = 500;
extern int TakeProfit = 500;
extern int BULevel = 300;
extern int TrailingStop = 300;
extern int Slip = 30;
extern int Magic = 123;
extern double Lots = 0.1;
//--- indicator parameters
input int InpFastEMA = 12; // Fast EMA Period
input int InpSlowEMA = 26; // Slow EMA Period
input int InpSignalSMA = 9; // Signal SMA Period
datetime t=0;
//+------------------------------------------------------------------+
//| Expert initialization function |
//+------------------------------------------------------------------+
int OnInit()
{
//---
//---
return(INIT_SUCCEEDED);
}
//+------------------------------------------------------------------+
//| Expert deinitialization function |
//+------------------------------------------------------------------+
void OnDeinit(const int reason)
{
//---
}
//+------------------------------------------------------------------+
//| |
//+------------------------------------------------------------------+
void PutOrder(int type,double price)
{
int r=0;
color clr=clrNONE;
double sl=0,tp=0;
if(type==1 || type==3 || type==5)
{
clr=Red;
if(StopLoss>0) sl=NormalizeDouble(price+StopLoss*Point,Digits);
if(TakeProfit>0) tp=NormalizeDouble(price-TakeProfit*Point,Digits);
}
if(type==0 || type==2 || type==4)
{
clr=Blue;
if(StopLoss>0) sl=NormalizeDouble(price-StopLoss*Point,Digits);
if(TakeProfit>0) tp=NormalizeDouble(price+TakeProfit*Point,Digits);
}
r=OrderSend(NULL,type,Lots,NormalizeDouble(price,Digits),Slip,sl,tp,"",Magic,0,clr);
return;
//---
}
//+------------------------------------------------------------------+
//| Check for open order conditions |
//+------------------------------------------------------------------+
void OpenPos()
{
double div1=iCustom(Symbol(),0,"DivOsMA",InpFastEMA,InpSlowEMA,InpSignalSMA,0,1);
double div2=iCustom(Symbol(),0,"DivOsMA",InpFastEMA,InpSlowEMA,InpSignalSMA,0,2);
double div3=iCustom(Symbol(),0,"DivOsMA",InpFastEMA,InpSlowEMA,InpSignalSMA,0,3);
//--- sell conditions
if(div1<div2 && div2>div3)
{
PutOrder(1,Bid);
}
//--- buy conditions
if(div1>div2 && div2<div3)
{
PutOrder(0,Ask);
}
//---
}
//+------------------------------------------------------------------+
int CountTrades()
{
int count=0;
for(int i=OrdersTotal()-1;i>=0;i--)
{
if(OrderSelect(i,SELECT_BY_POS,MODE_TRADES))
{
if(OrderSymbol()==Symbol() && OrderMagicNumber()==Magic)
{
if(OrderType()==OP_BUY || OrderType()==OP_SELL)
count++;
}
}
}
return(count);
}
//+------------------------------------------------------------------+
void Trailing()
{
bool mod;
for(int i=0; i<OrdersTotal(); i++)
{
if(OrderSelect(i,SELECT_BY_POS,MODE_TRADES))
{
if(OrderSymbol()==Symbol() || OrderMagicNumber()==Magic)
{
if(OrderType()==OP_BUY)
{
if(Bid-OrderOpenPrice()>TrailingStop*Point)
{
if(OrderStopLoss()<Bid-TrailingStop*Point)
{
mod=OrderModify(OrderTicket(),OrderOpenPrice(),Bid-TrailingStop*Point,OrderTakeProfit(),0,Yellow);
return;
}
}
}
if(OrderType()==OP_SELL)
{
if((OrderOpenPrice()-Ask)>TrailingStop*Point)
{
if((OrderStopLoss()>(Ask+TrailingStop*Point)) || (OrderStopLoss()==0))
{
mod=OrderModify(OrderTicket(),OrderOpenPrice(),Ask+TrailingStop*Point,OrderTakeProfit(),0,Yellow);
return;
}
}
}
}
}
}
}
//+------------------------------------------------------------------+
void BU()
{
bool m;
for(int i=0; i<OrdersTotal(); i++)
{
if(OrderSelect(i,SELECT_BY_POS,MODE_TRADES))
{
if(OrderSymbol()==Symbol() || OrderMagicNumber()==Magic)
{
if(OrderType()==OP_BUY)
{
if(OrderOpenPrice()<=(Bid-(BULevel+20)*Point) && OrderOpenPrice()>OrderStopLoss())
{
m=OrderModify(OrderTicket(),OrderOpenPrice(),OrderOpenPrice()+20*Point,OrderTakeProfit(),0,Green);
return;
}
}
if(OrderType()==OP_SELL)
{
if(OrderOpenPrice()>=(Ask+(BULevel+20)*Point) && OrderOpenPrice()<OrderStopLoss())
{
m=OrderModify(OrderTicket(),OrderOpenPrice(),OrderOpenPrice()-20*Point,OrderTakeProfit(),0,Green);
return;
}
}
}
}
}
}
//+------------------------------------------------------------------+
//| OnTick function |
//+------------------------------------------------------------------+
void OnTick()
{
if(t!=Time[0])
{
OpenPos();
t=Time[0];
}
if(BULevel>0) BU();
if(TrailingStop>0) Trailing();
//---
}
//+------------------------------------------------------------------+
datetime NY=D'2015.01.01 00:00'; // время наступления 2015 года
datetime d1=D'1980.07.19 12:30:27'; // год месяц день часы минуты секунды
datetime d2=D'19.07.1980 12:30:27'; // равнозначно D'1980.07.19 12:30:27';
datetime d3=D'19.07.1980 12'; // равнозначно D'1980.07.19 12:00:00'
datetime d4=D'01.01.2004'; // равнозначно D'01.01.2004 00:00:00'
AM2